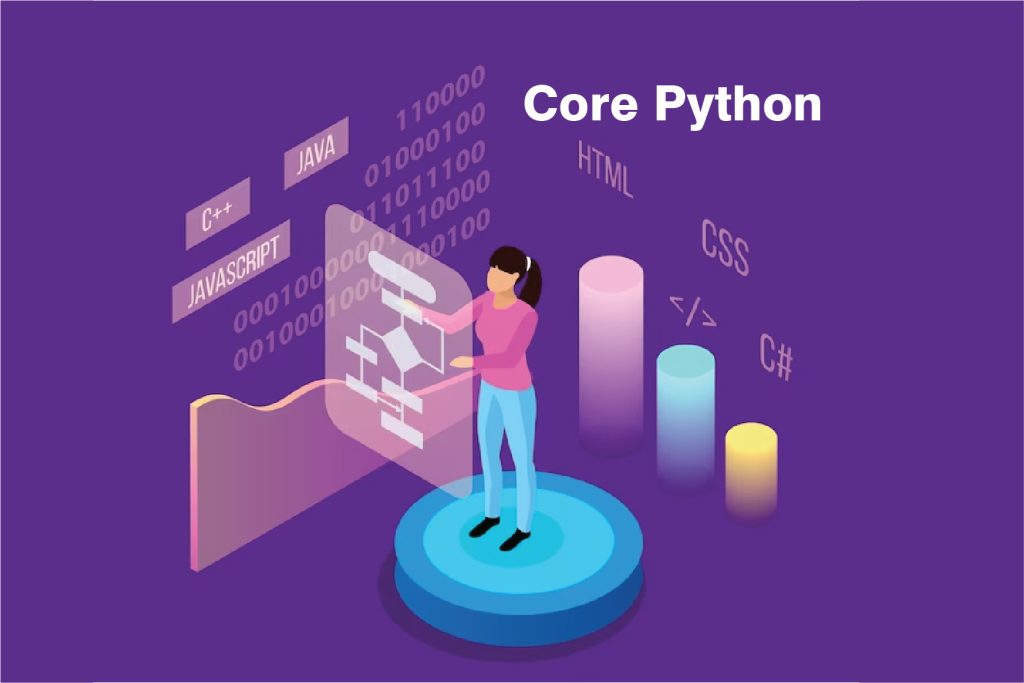
What are Python Classes?
Speaking about Python development as such, the Python classes are the general contractors who build solid and reliable code assets. Picture a class as a blueprint that, on a very detailed level, specifies the attributes and methods that all objects created by the class will have.
It is like the cookie cutter that makes the cookies awesome. The lesson goes over the qualities the product has (for instance ‘diameter’ and ‘ingredients’) and its basic methods. g. Implement these two basic processes i. e. ‘bake’ and ‘cool’ on each of the cookies that you will come up with. The goodness of every treat (object) will differ in its characteristics of size and flavor. In any case, all of them will have the same characteristics due to the class.
At this point, methods come into play. These can be viewed as features of the class that are specifically designated to handle the data (attributes) of the object. The ‘bake’ method would have the ‘temperature’ attribute increased to a higher level and the ‘cool’ method may lower it.
The classes and methods perform the role of the melody and harmony of a song. By this, you acquire the highest level of organization and reusability. Python Classes can keep your objects consistent while methods are precise representations of object behavior, thus improving the efficiency of the code. This way complex projects can be made easier to manage where you can write your code in a clear and maintainable manner.
Consequently, in the next Python programming encounter know that you are confident of classes – the organizers behind these real programming objects that are always ready to meet any challenge you provide to them.
Python classes methods
In the world of Python programming, Python classes methods are the cornerstones of building powerful and organized code. Here’s a breakdown of their key roles:
Classes: The Blueprints
Imagine a class as a blueprint for creating objects. It defines the attributes (variables) and methods (functions) that all objects of that class will possess. Think of it as a cookie cutter for creating similar cookies (objects) with specific characteristics.
For instance, you might create a Car class with attributes like model, color, and year. Methods within this class could be accelerated (), brake(), and turn().
Methods: The Actions
Methods are essentially functions defined within a class. They operate on the data (attributes) of a specific object. They dictate how the object behaves and interacts with the world.
Continuing with the Car example, the accelerate() method would increase the car’s speed attribute, while the brake() method would decrease it.
There are different types of methods:
- Instance Methods: These operate on a specific object instance. For example, car1.accelerate() would accelerate a particular car object (car1).
- Class Methods: These are utility functions associated with the class itself. They typically don’t operate on specific objects but might be used for tasks like creating new objects.
- Static Methods: These are helper functions that don’t directly interact with the class or its objects. They provide general utility within the program’s context.
By effectively utilizing classes and methods, you can structure your code efficiently and create reusable, maintainable programs. Classes ensure consistency and organization, while methods define the functionality of your objects, bringing your programs to life. Following the Python programming world, classes, and objects allow us to obtain powerful class architecture that is well structured.
Python classes and functions
In the programming world, Python classes and functions become one in the formation of inventive and logical programs.
Python Functions: The Building Blocks
A function is a reusable code block that completes some tasks. They can take two things: inputs (parameters) and outputs (return values) hence logical divide in the code can improve the code and prevent repetition.
Imagine the function calculate_area() calculating the area of the rectangle given its length and width as inputs and returning the result. The function can be called as many times as you need and use different values to calculate the areas of rectangles with varying sizes.
Python Classes: The Blueprints
The class acts as a pattern for making object instances. They determine the fields (variables) and methods (functions) that will be common to all objects of that class. Consider it as a blueprint for baking cookies (objects) that contain their ingredients (attributes) as well as baking instructions (methods).
As an example, you might make the Dog class with the attributes called name, breed, and age. It could have such methods as bark(), wag_tail(), and play_fetch().
The Beautiful Collaboration
Python Classes and functions collaborate to perform functions correctly. Methods, which declare the behavior of an object, are functions that reside within the class body. They act through the data (attributes) of that unique object instance.
Taking a Dog as an example, the bark() method would print a barking sound, and it would access the dog’s name attribute to mention the dog’s name when barking. (e. g., “Fido barks!”).
The teaming power
The mix of Python classes and functions provides for this level of high organization and the reusability of your code functionality gives modularity while classes apply structure and consistency to objects.
Python classes and object exercises
Attending Python classes and object exercises will help you excel in programming and become proficient in coding
Simulating the Real World:
In many cases, exercises include the task of building Python classes that model real-world objects. You could create a Book class that has attributes such as title, author, and genre and methods like lend() and return(). Through this method, you transition from theory to actual code.
Experimenting with Functionality:
Exercises give you a chance to use Python Classes and Objects in different ways. How about writing methods to calculate the area of a Rectangle object or classifying a shopping cart with methods to add and remove items? Such tasks boost your critical thinking and ability to employ classroom concepts.
Building Interactive Programs:
One of the exercises asks us to build programs that make use of Python classes and objects. Consider designing a straightforward game with a Player class which includes attributes like health and experience. Through this work, you will be challenged to think about the interaction between objects and user input thereby preparing you for actual application development.
Testing and Debugging:
Exercises typically do tests of units to make sure your classes and objects work as expected. This promotes proper coding etiquette and allows you to diagnose and fix errors in your programs, an invaluable skill for any developer.
Practice Makes Perfect:
The great thing about exercise is that you can start with the basics and then gradually move on to more complex activities. From initial Student and Book classes to even more complex simulation scenarios, the list goes on and on. Continuous practice is essential to becoming accomplished in OOP in Python.
Through active involvement in Python classes and object practice, the theoretical knowledge will be turned into practical skills and you will finally become a versatile Python programmer who can solve real-life challenges.
Python Classes Constructor
In the world of Python’s object-oriented programming, different Python classes constructor is an indispensable part of the process of creating objects.
The Birthplace of Objects: The Constructor
Some Python classes constructor has a particular method called the __init__(). This method plays the role of the constructor which automatically invokes whenever you create a new object (instance) of that class. Think of it like the assembly line where your objects are produced and initialized in their initial properties.
Initializing Attributes:
The main duty of the constructor is to initialize object attributes (variables). These attributes distinctly explain the individual features of each object. Within the __init__() method, you can assign values to the object’s attributes by using the self keyword. For example, you may have a Car class that has model and color attributes. In the constructor, you can take arguments like model, color, etc from the object creation process and assign them to the object’s attributes using self. model = self and model. color = color.
Customizing Object Creation:
In constructors, you can customize the way objects are created. You can then specify the initial values of attributes while creating the object by defining the parameters (arguments) within the __init__() method. This will allow you to have the right data objects set up from the very beginning.
Beyond Basics:
The construction is primarily for initialization purposes and sometimes also performs additional tasks like argument validation checks and consistency checks. It guarantees that the object is constructed properly.
Understanding Constructors is Key:
Understanding the principle of constructors is the key component of object-oriented programming in Python. They let you configure your objects in a controlled way, which makes your code more organized and easier to maintain. The use of constructors efficiently results in well-defined objects that are ready to play their roles within your programs. In the fantastic realm of Python classes, properties are one of the tools, which provide a way to manage and control access to an object’s attributes.
Beyond Simple Attributes:
Constant classes in Python are just variables accessed directly through the dot notation (object. attribute). By all means, they introduce a new level of control where you can define the getters, setters, and even removes for properties.
Getters, Setters, and Deleters:
Properties are created using the property() function that is built in. Such a function may receive arguments that would define getter, setter, and deleter methods corresponding to the property. The getter method will be invoked anytime you attempt to access the property using dot notation (object. property_name). Similarly, the setter method is called when you fix a new value to the property (object. property_name = value). Deleters enable you to specify the way you want an attribute to be deleted (del object. property_name, for example).
Conclusion
Finally, Python classes enable programmers to unleash the boundless possibility of programming. You’ll be able to learn how to structure your code using classes and objects and in return tackle problems that require modular and maintainable programming solutions. This makes you believe you can deal with difficult situations straightforwardly and confidently. Regardless of what field of information technology you are interested in – web development, data analysis, or the intriguing and always expanding field of machine learning – a class in Python will define the core skills you need to flourish in the ever-changing tech world. Therefore, taking the step and roaming Python classes can take you to the discovery of object-oriented programming with Python.